Nodejs快速接入GraphQL
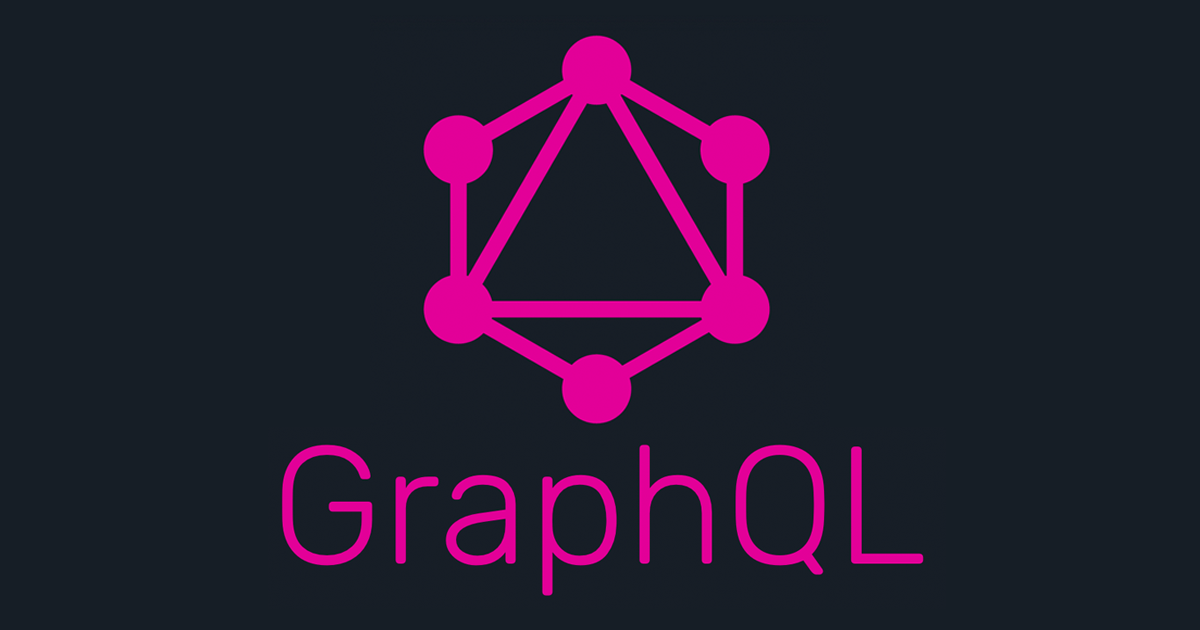
1. GraphQL简介
1.1 GraphQL
GraphQL 既是一种用于 API 的查询语言,也是一个满足你数据查询的运行时工具。 GraphQL 对 API 中的数据提供了一套易于理解的完整描述,使得客户端能够准确地获得它需要的数据,而没有任何冗余,让前后端开发联调效率更高,也让 API 更容易更新和维护。
使用效果类似:
GraphQL的好处不仅限于此,总结如下:
- 请求你所要的数据,不多不少, 减少API请求的数据冗余和请求冗余
- 具有灵活且强类型的schema,方便定义接口协议,且具有强类型校验
- 可提升联调、开发效率,API易于更新和维护
1.2 Apollo Graphql组件
Apollo GraphQL 是基于 GraphQL 的全栈解决方案集合。它开源提供了graphql前端到后端的一整套解决方案,包括 Apollo Client 和 Apollo Server等。让 GraphQL 使用更加简单,降低了使用门槛。
Apollo Server提供node端开箱即用的框架中间件,涵盖express和koa;Apollo Client同样也有对应的前端react、angularjs、vue的组件,可以直接引入使前端调用更加便捷。
2. demo项目介绍
2.1 技术栈
demo项目技术栈为nodejs(koa) + vue + webpack + mongodb,后端接口用koa2实现,前端使用框架vue实现,构建工具webpack,数据库使用mongodb。
项目目录结构如下所示:
├─controller // 后端服务接口实现
├─graphql // graphql schema定义以及resolver实现
├─routes // 后端接口路由
├─vue-dist // web前端build生成静态目标文件
└─web // web前端源文件以及webpack配置文件
└─src
├─assets
├─components
├─router
└─views
2.2 页面展示
首页(书籍列表):
书籍详情页:
页面结构非常简单,首页是一个书籍列表页面,点击书籍名称跳转到书籍详情页,详情页包含书籍对应名称、图片、作者名称、作者出版的其他书籍信息。
2.3 问题分析
如此简单的前端页面,看起来没有什么问题,但分析接口调用就能发现restful接口比较普遍存在的一些问题。
-
- API接口请求数过多
如上图所示,详情页面当前书籍的信息和作者出版的其他书籍信息,一共要调用三次不同的API接口来获取数据(通过书籍id获取当前书籍信息->根据作者名称获取作者出版书籍id->根据书籍id获取书籍相关信息)。
- API接口请求数过多
-
- 接口返回字段冗余
书籍列表页只需要展示书籍id、书籍名称和图片,然而实际接口返回会有很多冗余的字段:
部分项目中,同一个restful接口可能会提供给多个页面或终端使用,而不同调用方需要的数据内容不尽相同,后端不可能每次都针对不同调用方新增接口,所以只能最大程度涵盖所需要的字段。
- 接口返回字段冗余
-
- 字段校验问题
开发过程中比较耗时和容易出差错的地方,很大概率都是前后端联调。后端开发提供接口文档给前端开发人员,前端根据接口文档来mock或直接请求接口进行联调。联调主要在于对齐字段名称和字段类型,如果请求中字段错误导致BUG,而后段接口提示又比较模糊就会比较难定位问题;而如果接口有变动,后端通过接口文档更新维护并通知前端,成本也是比较高的。
- 字段校验问题
注:本demo项目的案例并不一定合理,但以上是restful接口容易遇到的一些问题。
3. 接入GraphQL
以上demo项目的问题基本都能用graphql来解决,本文使用Apollo GraphQL的一系列组件实现快速接入。
3.1 定义schema
GraphQL官方规范使用schema来定义数据结构和类型,以及请求的类型。我们使用schema定义语言(SDL)来定义服务端将返回的数据对象,指定对象的字段的类型和字段名。GraphQL server的请求处理和字段校验都依赖于定义好的schema。例如本demo所用到的接口对象可定义如下 (schema.js):
// gql标签函数,用于解析查询模版字符串生成graphql标准的AST以便组件能进行统一处理
const { gql } = require("apollo-server-koa");
// Construct a schema, using GraphQL schema language
const typeDefs = gql`
type Query {
bookList(names: [String]): [BookInfo]
bookDetail(id: String): BookDetail
}
type BookInfo {
_id: String!
name: String
author: String
isbn: String
url: String
img: String
}
type AuthorInfo {
_id: String!
name: String
address: String
company: String
books: [BookInfo]
}
type BookDetail {
bookInfo: BookInfo,
authorInfo: AuthorInfo
}
`;
module.exports = {
typeDefs
};
以上定义了三个请求接口(type Query),两个数据对象,并定义了对应的数据字段和类型;例如 bookInfo(id: String!): BookInfo
定义了请求接口,请求参数为String类型的id,响应为定义的数据对象BookInfo。
3.2 实现resolver
resolver是决定如何为schema填充数据的函数,填充数据可以是从数据库直接读取,也可以是调用其他的API。类似于接入graphql之前demo项目的controller实现;不同的是resolver函数中不需要指定返回的数据字段,graphql server会根据请求自动过滤和校验字段。如下代码为根据schema中定义的三个query实现的resolver函数:
// Provide resolver functions for your schema fields
const monk = require('monk');
const db = monk('localhost/library');
const books = db.get('books');
const authors = db.get('authors');
const resolvers = {
Query: {
// 根据书名查询书籍信息
bookList(parent, args, context, info) {
const names = args.names;
let params = Array.isArray(names) ? {
name: {
$in: names
}
} : {};
return books.find(params, {
sort: {
img: -1
}
});
},
// 根据id查询书籍详情
async bookDetail(parent, args, context, info){
const id = args.id;
const bookInfo = await books.findOne({
_id: id
});
const authorInfo = await authors.findOne({
name: bookInfo.author
});
let params = {
name:{
$in: authorInfo.books
}
}
const otherBookInfos = await books.find(params);
return {bookInfo, authorInfo:{
...authorInfo,
books:otherBookInfos
}};
}
},
};
module.exports = {
resolvers
};
3.3 创建graphql server
在app.js中引入ApolloServer的koa中间件,同时引入之前定义的schema和resolvers函数,使用中间件即可创建graphl server处理graphql请求,且可与koa服务监听同一个端口:
const { ApolloServer } = require("apollo-server-koa");
const { typeDefs } = require("./graphql/schema");
const { resolvers } = require("./graphql/resolvers");
// 创建graphql server
const server = new ApolloServer({
typeDefs,
resolvers,
debug: false
});
app.use(server.getMiddleware());
app.listen({ port: 8000 }, () =>
console.log(`🚀 Server ready at http://localhost:8000${server.graphqlPath}`)
);
自此,启动nodejs服务成功后,graphql server也启动成功了,apollo server还贴心地集成了GraphQL Playground方便开发者调试graphql请求:
3.4 调用graphql接口
前端代码中调用graphql接口同样非常简单,三大框架分别都有对用的组件可以直接使用:@apollo/react-hooks、vue-apollo、Angular Apollo。本文demo虽然使用的是vue框架,但前端没有集成vue-apollo组件,而是采用了比较通用的调用方式:
web/src/main.js
import Vue from 'vue'
import App from './App.vue'
import router from './router'
import ApolloClient from 'apollo-boost';
import { InMemoryCache } from 'apollo-cache-inmemory';
import { HttpLink } from 'apollo-link-http';
// 提供客户端通过http链接请求graphql接口
const httpLink = new HttpLink({
// 可增加请求选项和 http headers
credentials: 'same-origin'
});
const client = new ApolloClient({
httpLink,
cache: new InMemoryCache() // 处理graphql本地缓存的组件
});
Vue.prototype.graphqlClient = client;
Vue.config.productionTip = false;
new Vue({
router,
render: h => h(App)
}).$mount('#app')
home.vue (书籍列表页请求graphgql部分代码):
import { gql } from "apollo-boost";
export default {
name: "List",
data() {
return {
bookList: []
};
},
created() {
this.initBookList();
},
methods: {
async initBookList() {
// 查询所有书籍列表
// let { bookList } = await this.postFetch("/fetchBookList", {});
// this.bookList = bookList;
this.getGraphqlBookList().then(res=>{
let bookList = res.data.bookList;
this.bookList = bookList;
})
},
// graphql接口查询书籍列表
getGraphqlBookList() {
return this.graphqlClient
.query({
query: gql`
query GetBookList {
bookList {
_id
name
img
}
}
`,
fetchPolicy: 'network-only' // default 'cache-first'
})
}
}
};
Detail.vue (书籍详情页请求graphgql部分代码):
import { gql } from "apollo-boost";
export default {
name: "Detail",
data() {
return {
bookId: "",
bookInfo: {},
publishBooks: []
};
},
created() {
// this.fetchDetailInfo();
this.getGraphqlBookDetail().then(res=>{
console.log(res)
let bookDetail = res.data.bookDetail;
this.bookInfo = bookDetail.bookInfo;
this.publishBooks = bookDetail.authorInfo.books;
});
},
methods: {
// graphql接口查询书籍列表
getGraphqlBookDetail() {
this.bookId = this.$route.params.id;
return this.graphqlClient.query({
query: gql`
{
bookDetail(id: "${this.bookId}") {
bookInfo {
name
img
isbn
author
}
authorInfo {
books {
name
img
isbn
}
}
}
}
`,
fetchPolicy: "network-only" // default 'cache-first'
});
}
}
};
请求graphql接口时均可以指定需要的字段,graphql server会对查询参数进行处理,只响应返回需要的字段。另外,apollo-cache-inmemory是用于graphql本地缓存管理的组件,请求时可指定 fetchPolicy 缓存策略,本文不深入这块的介绍,感兴趣可自行实践。
4. 效果对比
4.1 API 响应字段
fetchBookList为接入graphql前请求书籍列表数据,graphql则只返回需要的字段,由于响应字段数不同,流量消耗也不同:
4.2 API请求数量
API请求的数量对比,比较明显在本文demo的场景下,书籍详情页的数据请求由之前的三次API请求变为了一次:
4.3 字段校验
如下图,graphql对于字段名称和类型会有严格的校验,校验失败会返回详细的错误分类和错误栈信息:
而未使用graphql的情况下,若接口字段有变动而前端没有及时更新,往往会直接呈现为页面BUG,且难以定位。
本文demo代码均在:
https://github.com/xdevilj136/graphql-koa-demo